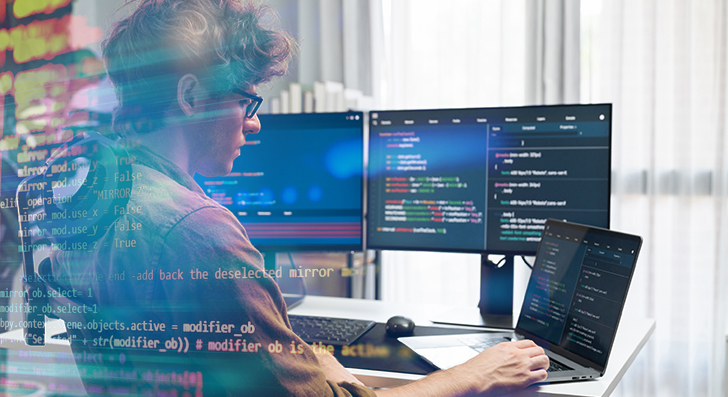
Scalability implies your software can manage development—more buyers, far more info, and much more traffic—without breaking. As a developer, developing with scalability in your mind saves time and worry later on. Right here’s a transparent and useful guideline to assist you to commence by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your system from the beginning. Many apps are unsuccessful after they mature rapidly because the initial structure can’t manage the additional load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Get started by developing your architecture to be versatile. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These patterns split your application into smaller, independent pieces. Every module or provider can scale By itself without affecting The entire process.
Also, give thought to your database from day just one. Will it need to handle 1,000,000 end users or merely 100? Pick the right kind—relational or NoSQL—determined by how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further vital stage is to prevent hardcoding assumptions. Don’t produce code that only works under existing disorders. Consider what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design patterns that support scaling, like message queues or occasion-driven techniques. These aid your app handle far more requests with no receiving overloaded.
Once you Construct with scalability in mind, you are not just planning for achievement—you are decreasing foreseeable future head aches. A nicely-prepared process is simpler to maintain, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the appropriate Database
Choosing the ideal databases is actually a key Element of constructing scalable programs. Not all databases are built the exact same, and utilizing the Improper one can sluggish you down or perhaps cause failures as your application grows.
Begin by being familiar with your knowledge. Is it remarkably structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good in shape. They're potent with associations, transactions, and regularity. Additionally they aid scaling procedures like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your facts is more versatile—like person activity logs, item catalogs, or files—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your read through and write patterns. Will you be doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for temporary information streams).
It’s also sensible to think ahead. You may not want Innovative scaling capabilities now, but deciding on a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Stay clear of avoidable joins. Normalize or denormalize your data based on your access patterns. And usually keep track of database overall performance as you develop.
In a nutshell, the best databases is dependent upon your app’s construction, speed needs, and how you expect it to mature. Choose time to select wisely—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Construct efficient logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Resolution if a simple a person performs. Keep your capabilities quick, focused, and simple to check. Use profiling tools to uncover bottlenecks—spots where your code can take also long to operate or uses an excessive amount memory.
Up coming, evaluate your database queries. These frequently gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid carrying out a lot of joins, Particularly throughout large tables.
Should you see exactly the same knowledge remaining asked for many times, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. Rather than updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to deal with 1 million.
In a nutshell, scalable applications are rapidly applications. Keep the code restricted, your queries lean, and use caching when wanted. These ways assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and much more site visitors. If almost everything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. Both of these equipment support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to a single server carrying out all of the work, the load balancer routes buyers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused rapidly. When users ask for exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quickly obtain.
two. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, improves pace, and makes your app extra effective.
Use caching for things which don’t change typically. And always be sure your cache is current when information does transform.
In short, load balancing and caching are basic but impressive resources. Together, they help your application tackle a lot more people, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need instruments that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t should invest in components or guess upcoming potential. When traffic increases, you are able to include a lot more assets with only a few clicks or instantly employing automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply solutions like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and all the things it really should operate—code, libraries, settings—into one device. This causes it to be uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and recover immediately when difficulties materialize. If you need your application to develop devoid of limits, start off utilizing these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, check here not repairing.
Observe Every thing
In case you don’t observe your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place challenges early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently before buyers even detect.
Monitoring is additionally helpful when you make variations. When you deploy a fresh function and find out a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of difficulty until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, monitoring helps you maintain your app reputable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to have a strong foundation. By building very carefully, optimizing correctly, and using the proper applications, you'll be able to Establish apps that expand effortlessly with out breaking under pressure. Get started little, Imagine large, and Make smart.